06. Actions
Let's start from the Dashboard View. Our Dashboard View displays a list of tweets and a menu.
We need to take a look at what is happening in this view. Let's determine what actions the app or the user is performing on the data - is the data being set, modified, or deleted?
Remember that in Step 4 of the Planning Stage, we determined that our store will look like this:
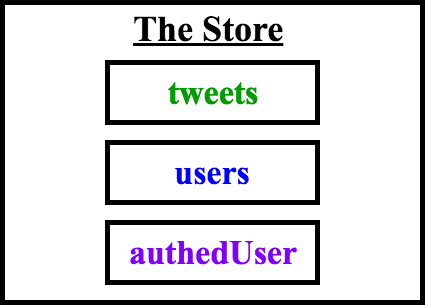
The Store contains a tweets property, a users property, and an authedUser property.
When the app loads, the Dashboard View is displayed. The Dashboard Component therefore needs to:
- get the tweets
- get the users
- get the authedUser
This data is stored in a database. For this view to load all of the tweets (including their author's avatars), we need to 1) get the tweets
and users
data from the database; and then 2) to pass that data into the component.
API Best Practices for React Apps
SOLUTION:
- The `componentDidMount()` lifecycle method
API Best Practices for React & Redux Apps
SOLUTION:
- From asynchronous action creators.
Remember how normal Action Creators return actions - simple Javascript objects that then go to all of our reducers? Making an API request is an asynchronous action, so we cannot just send a plain Javascript object to our reducers. Redux middleware can gain access to an action when it's on its way to the reducers. We'll be using the redux-thunk
middleware in this example.
If the Redux Thunk middleware is enabled (which is done via the applyMiddleware()
function), then any time your action creator returns a function instead of a Javascript object, it will go to the redux-thunk
middleware.
Dan Abramov describes what happens next:
“The middleware will call that function with dispatch method itself as the first argument…The action will only reach the reducers once the API request is completed.
It will also “swallow” such actions so don't worry about your reducers receiving weird function arguments. Your reducers will only receive plain object actions—either emitted directly, or emitted by the functions as we just described.”
Here's what a thunk action creator looks like:
function handleInitialData () {
return function (dispatch) {}
}
Which is equivalent to this in ES6:
function handleInitialData () {
return (dispatch) => {}
}
Now, we need to give our components access to the data that came in. In other words, we need to populate the store with tweets
and users
.
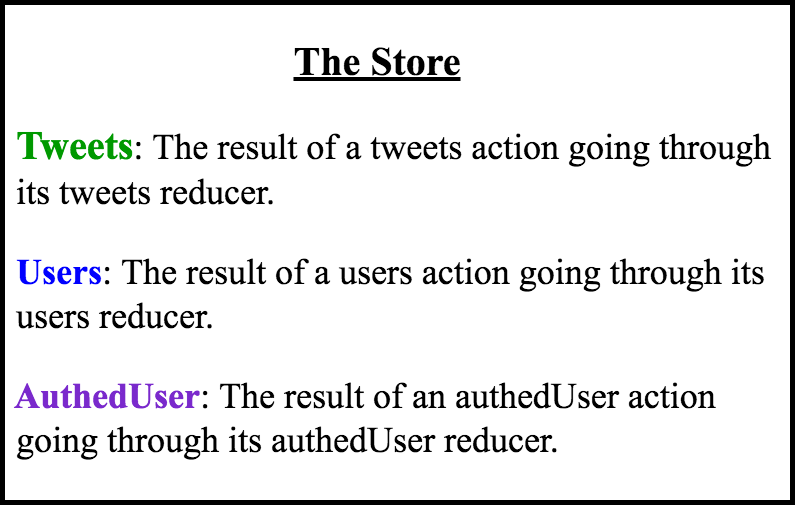
The Model of Our Store
The tweets slice of the state in the store will be modified by actions that go through the tweets reducer.
The users slice of the state in the store will be modified by actions that go through the users reducer.
And, similarly, the authedUser portion of the state in the store will be modified by actions that go through the authedUser reducer.
L705 First Actions V1
L729 Authorized User Action V1